Here we want to add some server side Java logic that will update the date of renegotiation of the contract. In our exemple it will simply set date of creation + three months, but you can think of integrating in your Java class a connection to an external webservice or an ERP.
Prerequisites
- A document type Contract created at the previous step
- Eclipse 4.3 for JEE developers (Kepler)
- Java 7
- A Nuxeo SDK: Nuxeo Platform 6.0 SDK (LTS).
Step 1 - Download Nuxeo IDE
From Eclipse Marketplace
This procedure can only be used for Nuxeo 6.0 and later versions. For Nuxeo 5.8, please refer to the installation from the Eclipse Menu.
- In Eclipse, go into the Help, Eclipse Marketplace menu.
- The Eclipse Marketplace window opens.
- Search for Nuxeo, select Nuxeo IDE and click on the Install button.
- Nuxeo IDE and Nuxeo Shell are automatically selected and downloaded.
- When Nuxeo IDE and Nuxeo Shell are downloaded, click on the Next button.
- Accept license when prompted. Installation begins. After a few seconds, a security warning is prompted.
- On the security warning window, click on OK.
Installation continues.
- Restart Eclipse when prompted.
Nuxeo IDE is installed. A new button is available in the Eclipse toolbar:
From Eclipse Menu
- Open the install dialog from the Eclipse menu: Help > Install New Software...
- Click on Add... button and enter the Update Site URL (stable for the latest released version, or development for a development snapshot) and name and click the OK button.
- Update site for latest Nuxeo 5.8 compatible version: http://community.nuxeo.com/static/nuxeo-ide/releases/1.2.4.R12x_v20141120_1114/site/
- Update site for latest Nuxeo IDE snapshot version: http://community.nuxeo.com/static/nuxeo-ide/dev/site/
- Update site for latest Nuxeo IDE stable version: http://community.nuxeo.com/static/nuxeo-ide/stable/site/
- To get latest Nuxeo IDE from QA: http://qa.nuxeo.org/jenkins/job/nuxeo-ide-master/ws/nuxeo-ide/sites/org.nuxeo.ide.site/target/site/
- If nothing appears, uncheck the Group items by category box.
Check both Nuxeo IDE and Nuxeo Shell and click Next.
Follow the wizard: accept license when prompted and click Next until the software is downloaded.
- Restart Eclipse when prompted.
Nuxeo IDE is installed. A new button is available in the Eclipse toolbar:
Step 2 - Register a Nuxeo Online Services Account
To configure a Nuxeo Online Services account:
- Open Eclipse preferences and go to Nuxeo > Nuxeo Connect. The Host name is already filled in with Nuxeo Connect address.
- Type your Nuxeo Online Services login and password and click on the Connect button.
The lists of Studio projects associated to your account is displayed.
- Click on OK. The Preferences window closes. You can now go to the Studio view to browse your Studio customizations.
Step 3 - Register a Nuxeo SDK
- In the Nuxeo SDK preference page (Eclipse Menu -> Window -> Preferences -> Nuxeo -> Nuxeo SDK), click on Add....
- Select the directory where you unzipped your Nuxeo distribution.
Be sure to select the right directory: the one containing the
bin
andnxserver
directories. - Check the added Nuxeo SDK and click on the OK button to finish. Checking the box at the left of the SDK is required to mark it as the current Nuxeo SDK used in the Eclipse Workspace.
Step 4 - Implement an Operation
Here we want to create an operation that indicates the date of renegotiation of a contrat by fetching the date of creation of the document and adding three months to it. An operation is a Java class in which you can put some logic and that can be exposed in Studio for being easily reused when implementing your business logic. See the Automation section for more information about operations.
Create your Nuxeo Plugin Project
- Click on
> Nuxeo Plugin Project and click on Next.
- Fill in the Create project wizard:
- Project: I will call it
ContractRemindProject
. - Root package:
org.nuxeo.sample
- Project: I will call it
- Click on Next.
- Fill in the Maven settings:
- Groupe Id:
org.nuxeo
- Artifact Id:
ContractRemindProject
- Artifact Version: 1.0-SNAPSHOT
- Parent Groupe Id:
org.nuxeo
- Parent Artifact Id:
nuxeo-addons-parent
- Parent version: 6.0
- Additional information:
ContractRemindProject
- Groupe Id:
- Click on Finish.
Coding your operation
- Click on
> Automation > Operation wizard and click on Next.
Fill in the Create operation wizard:
- Project: the box is already filled with
ContractRemindProject
. - Package:
org.nuxeo.sample.operation
. - Name: I will call it
ContractUpdater
. - Operation Id: I will use
ContractUpdater
too. - Category: select
CAT_DOCUMENT
. - Requires Seam Content: My operation does not need the Seam context so I will leave this box unticked.
- Operation Signature: Each operation accepts and returns something. For our operation, I will select document for both input and output.
- Iterable Operation: Ticked this box.
- Project: the box is already filled with
- Click on Finish. You should now have end up with something like this:
/**
* @author mlumeau
*/
@Operation(id=ContractUpdater.ID, category=Constants.CAT_DOCUMENT, label="ContractUpdater", description="")
public class ContractUpdater {
public static final String ID = "ContractUpdater";
@OperationMethod(collector=DocumentModelCollector.class)
public DocumentModel run(DocumentModel input) {
return null;
}
}
Time to file the skeleton and start coding. Here is the final result:
/**
* @author mlumeau
*/
@Operation(id = ContractUpdater.ID, category = Constants.CAT_DOCUMENT, label = "ContractUpdater", description = "")
public class ContractUpdater {
public static final String ID = "Sample.ContractUpdater";
private static final String CONTRACT_TYPE = "Contract";
private static final String CONTRACT_SCHEMA = "contract";
private static final String CONTRACT_START = CONTRACT_SCHEMA + ":start";
private static final String CONTRACT_REMINDER = CONTRACT_SCHEMA
+ ":reminder";
@OperationMethod(collector = DocumentModelCollector.class)
public DocumentModel run(DocumentModel input) throws ClientException {
if (!(CONTRACT_TYPE.equals(input.getType()))) {
throw new ClientException("Operation works only with "
+ CONTRACT_TYPE + " document type.");
}
Calendar start = (Calendar) input.getPropertyValue(CONTRACT_START);
Calendar reminder = (Calendar) start.clone();
reminder.add(Calendar.MONTH, 3);
input.setPropertyValue(CONTRACT_REMINDER, reminder.getTime());
return input;
}
}
Send the Operation in Studio
- Click on the Perspective button
at the top right corner of the Eclipse window. Two new tabs appear on the left menu.
- Click on the Export Operation button
on the Nuxeo Studio tab of the left menu.
- Select the Studio project in the drop down menu, and the Eclipse project to look for operation. Then click Next.
Step 5 - Deploy the Project on the Server
Creating a deployment profile
Here we want to create a new default profile, called "My Projects" that includes the ContractRemindProject
.
- Go into the Nuxeo Server view (on the bottom right).
- Click on the button
in the view toolbar. The button tooltip is "Select projects to deploy on server".
- Click on Add.
- Enter a name (in the right panel) for your deployment profile. Example: "My Projects”.
- In the left panel click on the newly created profile.
- In the right panel, check the projects that will be deployed with this profile.
- If you want the new profile to be the default deployment profile, check it.
- Click on the OK button.
- Here is what you should get:
Starting the server
To start the server:
- Click on the Run button in the toolbar (
).
- Wait a minute (or more) until the server starts.
When done (i.e when the Stop button is enabled), open a browser and login using the 'Administrator/Administrator' account.
You can open the browser by clicking the Browse button (
).
Step 6 - Create Your Chain in Nuxeo Studio
Step 1: Create an automation chain
- In Studio (left menu), Click on Automation > Automation Chains > New.
I will call it
ContractUpdater
. - Keep the Fetch > Context Document(s) operation and add your own operation that you will find in Document > ContractUpdater.
- Click on Save.
Step 2: Create an Event Handler
You know have to create an Event Handlers in order to call your operation when a contract is created.
- In Studio (left menu), Click on Automation > Event Handlers > New.
I will call it
Reminder
- Fill in the creation wizard:
- Events: select About to create.
- Current document has one of the types: select your document type Contract.
- Event Handler Execution: Choose your automation chain
ContractUpdater
.
- Click on Save.
Now, you can try it on your server by deploying your changes on your Nuxeo Platform instance, to do so:
In Eclipse, click on the Refresh button in the Nuxeo Server view (
).
Go back in the browser, and refresh the
http://NUXEO_SERVER/nuxeo/site/test
page.
You should end up with something like this:
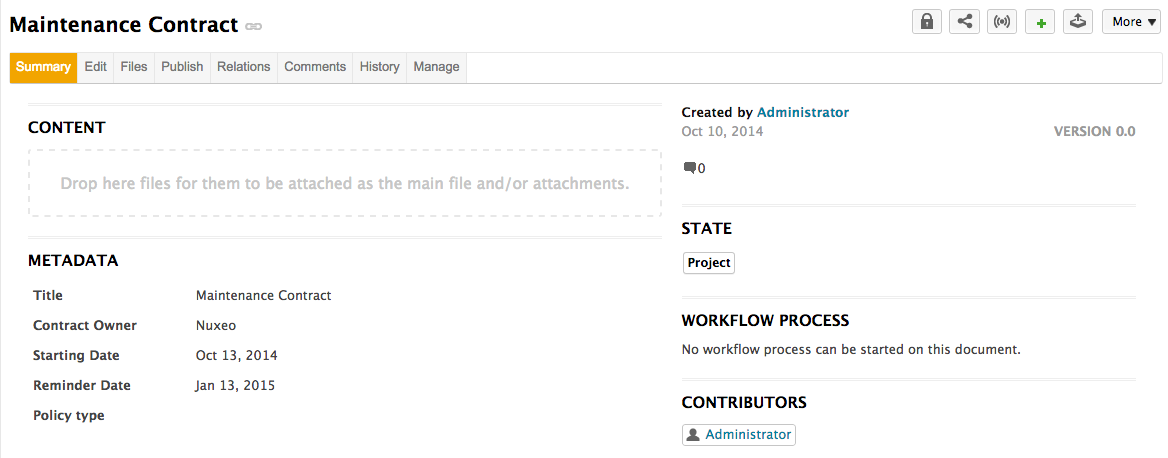
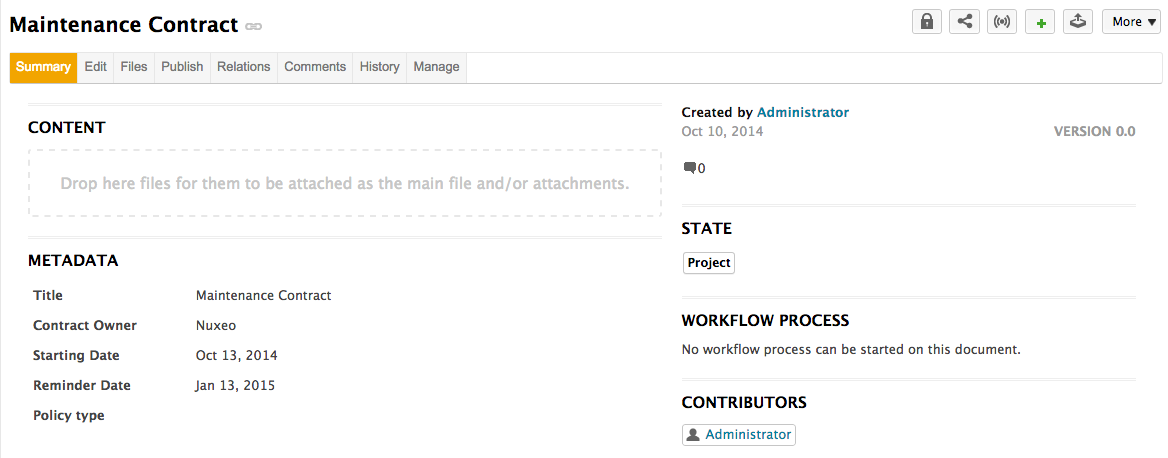
That's it! You are ready to develop on the Nuxeo Platform.