OAuth 2 is a protocol that allows an application to obtain access to the Nuxeo Platform on behalf of a user.
Nuxeo tries to stay very close to the "OAuth 2.0 Authorization Framework" RFC to ease client integration and be secure. Before going any further, because OAuth 2 has to make a lot of secure exchanges with clients using query parameters, you must ensure to have configured Nuxeo in HTTPS.
The RFC describes two endpoints:
- An Authorization endpoint used by the client to obtain authorization from the resource owner via user-agent redirection
- A Token endpoint used by the client to exchange an authorization code for a reusable access token
Installation
OAuth 2 is natively supported by the Nuxeo Platform, which means there is no bundle to install and no XML extensions required to enable it. An HTTP filter handles authentication in priority compared to the other filters run by the authentication chain.
Client Registration
Nuxeo allows you to register an OAuth 2 client by specifying an arbitrary name, a client ID, possibly a client secret and a list of redirect URIs. There are different ways to register a client:
Using JSF UI
- Go to the Nuxeo Platform web interface, then browse to the Admin Center > Cloud Services > Consumers tab.
- Provide a name, a client ID, possibly a client secret, at least one redirect URI and save.
Using the REST API
Use the directory
REST API endpoint to create a new entry in the oauth2Clients
directory, for instance with curl
:
curl -u Administrator:Administrator \
-H 'Content-Type: application/json' \
-X POST http://NUXEO_SERVER/nuxeo/api/v1/directory/oauth2Clients \
-d @- << EOF
{
"entity-type": "directoryEntry",
"directoryName": "oauth2Clients",
"properties": {
"name": "My App",
"clientId": "myApp",
"clientSecret": "secret",
"redirectURIs": "https://myapp.mydomain.com/nuxeo-auth-done",
"autoGrant": "true",
"enabled": "true"
}
}
EOF
Auto-grant
parameter, if checked, allows the OAuth 2 client to bypass the authorization phase, skipping the web page asking the user to allow the application to access the Nuxeo Platform assets. This is the case for the Mobile application.
The OAuth 2 endpoints are now ready to be used.
OAuth 2 Flows
OAuth 2 Authorization Grant Flow
Here is how Nuxeo handles the OAuth 2 flow to authorize your application to access a protected Nuxeo resource on behalf of a user.
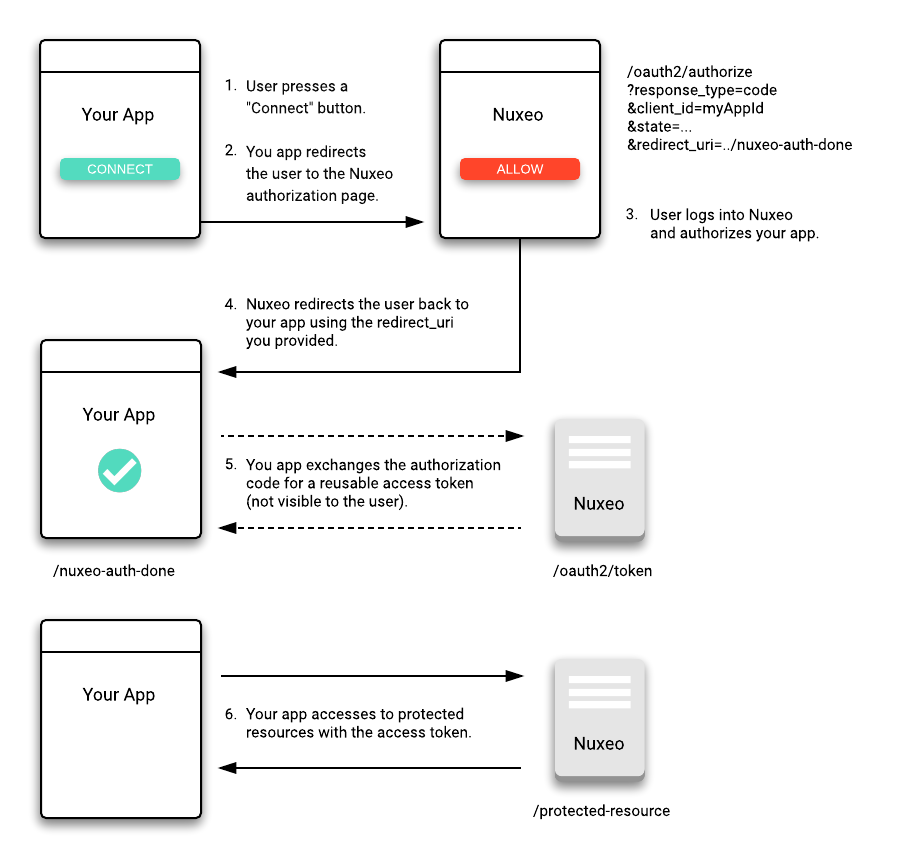
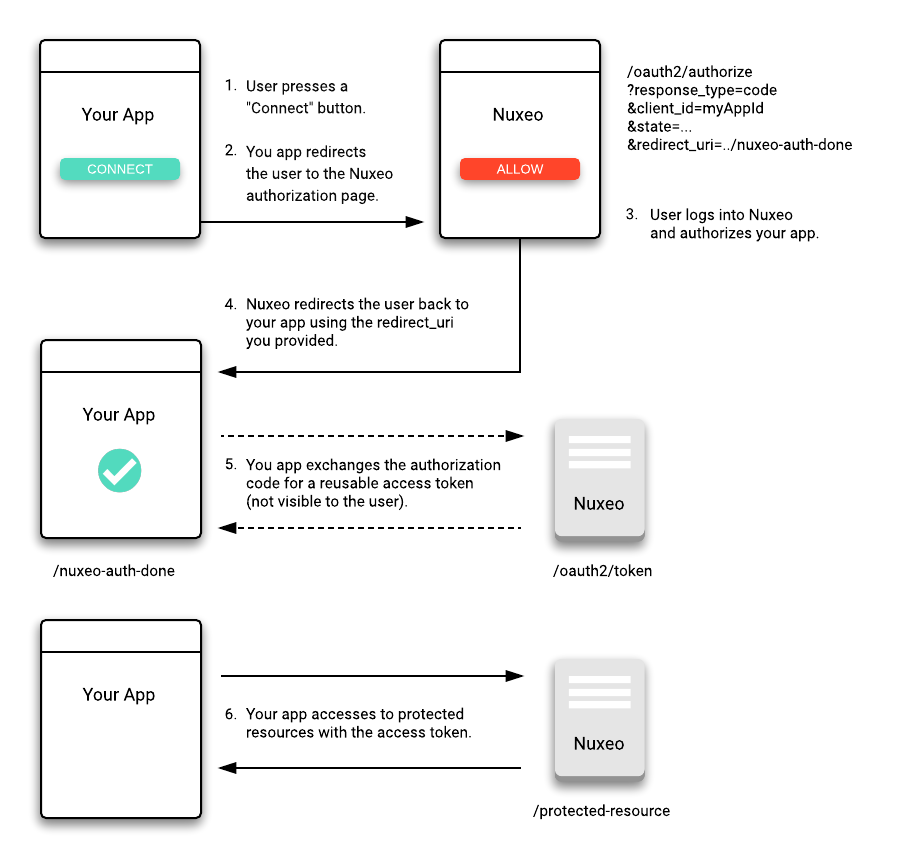
OAuth 2 JWT Flow
Here is how Nuxeo handles the OAuth 2 flow to authorize your application to access a protected Nuxeo resource thanks to a JSON Web Token (JWT) that contains identity and security information.
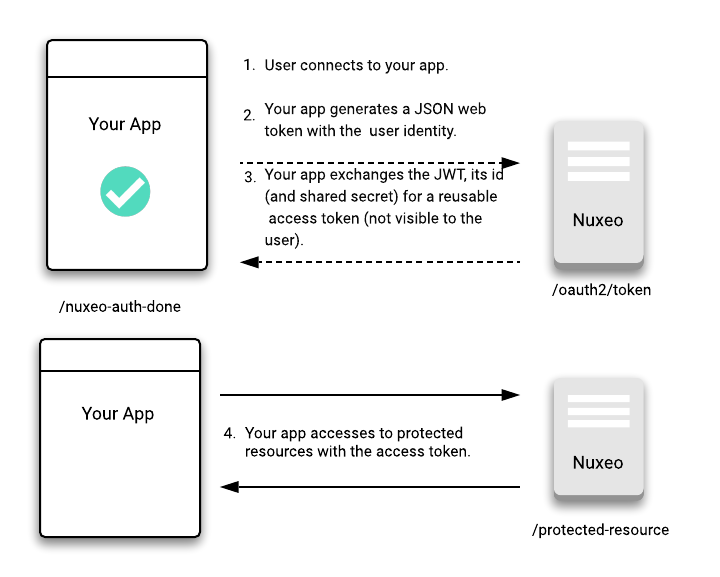
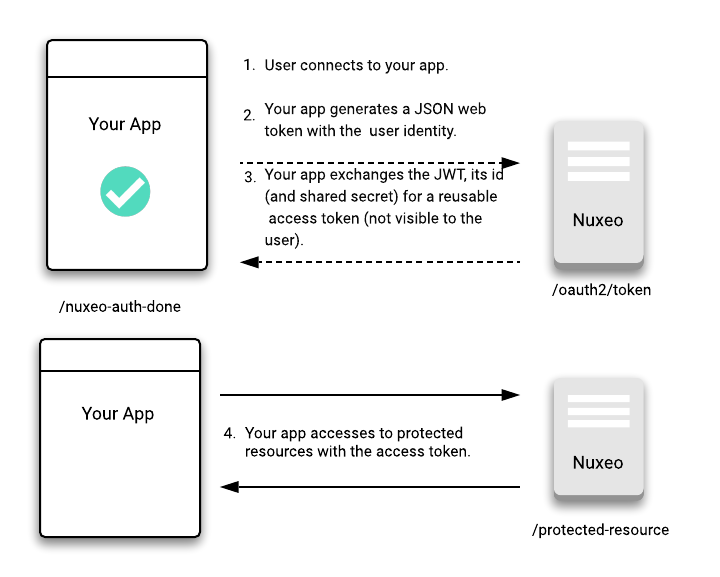
Authorization Endpoint
GET https://NUXEO_SERVER/nuxeo/oauth2/authorize?response_type=code&client_id=myApp
Query parameters:
Name | Type | Required | Description |
---|---|---|---|
response_type |
string | Yes | The value must be code for requesting an authorization code. |
client_id |
string | Yes | An enabled client identification. |
redirect_uri |
string | No | The absolute URI to return the user to after authorization is complete. |
state |
string | No | An opaque value used by the client to maintain a state between the request and the redirect callback. |
scope |
string | No | Ignored in our implementation. |
code_challenge |
string | No | A challenge derived from the code verifier, to be verified against later. |
code_challenge_method |
string | No | The code verifier transformation method: "S256" or "plain". |
Auto-grant
is not checked for the OAuth 2 client registered on the server, the user authorization is handled by accessing https://NUXEO_SERVER/nuxeo/oauth2Grant.jsp
. That lets you customize the way you want your users to let your application get authorized.
code_challenge
and code_challenge_method
parameters must be used with a public client, according to the "Proof Key for Code Exchange by OAuth Public Clients " RFC. For the code_challenge_method
, if the client is capable of using "S256", it must use "S256", as "S256" is implemented on the server.
Token Endpoint
Requesting an Access Token with an Authorization Code
POST https://NUXEO_SERVER/nuxeo/oauth2/token?grant_type=authorization_code&client_id=myApp&code=authorizationCode
Query parameters:
Name | Type | Required | Description |
---|---|---|---|
grant_type |
string | Yes | The value must be authorization_code for requesting an access token. |
client_id |
string | Yes | Must be the same as previously sent to the Authorization endpoint. |
client_secret |
string | No | The client's secret. |
code |
string | Yes | The authorization code received from the Authorization endpoint. |
redirect_uri |
string | No, only if sent to the Authorization endpoint. | Must be the same as previously sent to the Authorization endpoint. |
code_verifier |
string | No, only if code_challenge and code_challenge_method were sent to the Authorization endpoint. |
A high-entropy cryptographic random string using the unreserved characters [A-Z] / [a-z] / [0-9] / "-" / "." / "_" / "~" from Section 2.3 of RFC3986, with a minimum length of 43 characters and a maximum length of 128 characters. |
Response:
HTTP/1.1 200 OK
Cache-Control: no-cache
Content-Length: 176
Content-Type: application/json;charset=ISO-8859-1
{
"access_token": "A4zNYD1F7cp9l2UTL14pMT65qsyilgjZ",
"expires_in": 3599.0,
"refresh_token": "uFfVCD82NRlzeACoK6Fw09fvkYp6GmkuLs2UigconizFufNxIQZLd7btXLxzUzlB",
"token_type": "bearer"
}
Configuring Nuxeo for JWT Access
JWT authentication can be enabled on Nuxeo server if you set nuxeo.jwt.secret
within your nuxeo.conf
configuration file. The secret should be protected as a sensitive value.
Prepare the JWT Assertion
The JWT assertion makes use of the oauth2Clients
directory entry. The JWT bearer token must have several required fields to generate the OAuth 2 token.
Payload
The iss
(issuer) and sub
(subject) fields are required for JWT generation.
Example payload for JWT assertion:
{
"iss": "nuxeo",
"sub": "Nuxeo Subject Username"
}
Encode JWT
This example uses a node.js
package called jsonwebtokencli
to generate the encoded form. Equivalent functions can be found in the Java, Python, and JavaScript libraries.
The JWT secret value (nuxeo.jwt.secret
), timestamp
, and usage of the HS512
algorithm are required to properly encode a JWT token.
# Install JSON web token command line interface (once)
$ npm install -g jsonwebtokencli
# Encode the token with the above example for 'Administrator' user
$ echo '{"iss":"nuxeo","sub":"Administrator"}' | jwt -s yourSecretValueGoesHere -e --algorithm HS512 -t
# The response will be an encoded JWT value, like:
eyJhbGciOiJIUzUxMiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJudXhlbyIsInN1YiI6IkFkbWluaXN0cmF0b3IiLCJpYXQiOjE1ODA0MDEyODB9.uejUtvHXzXzKxedO9Jvvx2GK-4XmMgKW3DR2wbxIvxvC5c1z0atEfFhJJ9qqsMNkXvmm_n2CrcvwBhz3G0RXWg
Requesting an Access Token with a JWT
Use the JSON web token generated in the previous step as the assertion to generate the OAuth2 bearer token (abbreviated here).
POST /nuxeo/oauth2/token HTTP/1.1
Host: NUXEO_SERVER/nuxeo
Accept: application/json
Content-Type: application/x-www-form-urlencoded
grant_type=urn%3Aietf%3Aparams%3Aoauth%3Agrant-type%3Ajwt-bearer&client_id=myApp&client_secret=secret&assertion=eyJhbGci...
Input parameters:
Name | Type | Required | Description |
---|---|---|---|
grant_type |
string | Yes | The value must be urn:ietf:params:oauth:grant-type:jwt-bearer for requesting an access token. |
client_id |
string | Yes | The client id, registered in Nuxeo. |
client_secret |
string | No | The client's secret |
assertion |
string | Yes | The JSON web token |
The client ID and secret will be different than the JWT encoded secret.
Response:
HTTP/1.1 200 OK
Cache-Control: no-cache
Content-Length: 176
Content-Type: application/json;charset=ISO-8859-1
{
"access_token": "A4zNYD1F7cp9l2UTL14pMT65qsyilgjZ",
"expires_in": 3599,
"refresh_token": "uFfVCD82NRlzeACoK6Fw09fvkYp6GmkuLs2UigconizFufNxIQZLd7btXLxzUzlB",
"token_type": "bearer"
}
Refreshing an Access Token
POST https://NUXEO_SERVER/nuxeo/oauth2/token?grant_type=refresh_token&client_id=myApp&refresh_token=refreshToken
Query parameters:
Name | Type | Required | Description |
---|---|---|---|
grant_type |
string | Yes | The value must be refresh_token for refreshing an access token. |
client_id |
string | Yes | Must be the same as previously sent to the Authorization endpoint. |
client_secret |
string | No | The client's secret |
refresh_token |
string | Yes | A refresh token bound to the same client |
Response:
HTTP/1.1 200 OK
Cache-Control: no-cache
Content-Length: 176
Content-Type: application/json;charset=ISO-8859-1
{
"access_token": "jkCQ5cgDavJdXlIqWIWwivuhUA2heqBa",
"expires_in": 3599.0,
"refresh_token": "R9KzeZSnyo6ErTvYbPifehgsyIhDtdIhBp3XgJQKWcDo2ikZ8Vl2NlMV87d0KEIB",
"token_type": "bearer"
}
Authenticating Using an Access Token
Once you obtain an access token it can be used to access the protected Nuxeo resources with a request header or a query parameter. Like below using curl
:
curl -H "Authorization: Bearer ACCESS_TOKEN" https://NUXEO_SERVER/nuxeo/api/v1/path/default-domain
curl https://NUXEO_SERVER/nuxeo/api/v1/path/default-domain?access_token=ACCESS_TOKEN